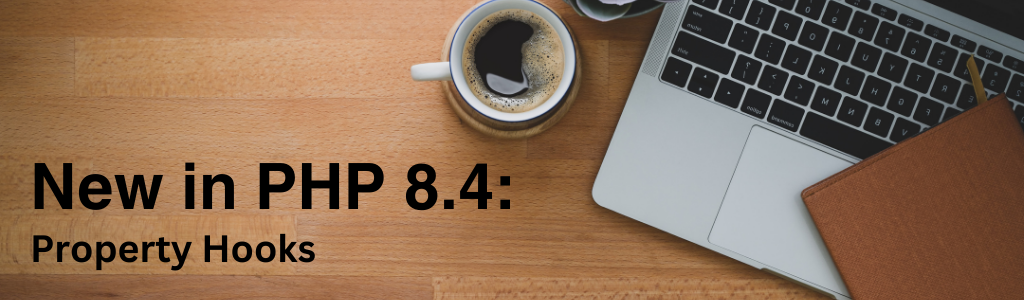
New in PHP 8.4: Property Hooks
Scott Keck-Warren • January 5, 2025
One of the new features added to PHP in the 8.4 release was the ability to define property hooks in our classes. Using property hooks we can override the default set
and get
operations to have it do something truely unexpected.
For example, in the class below we've defined a $fullName
property and we added a get
and set
property hook that allow us to interact with the $first
and $last
properties "magically".
<?php
class User
{
public function __construct(public string $first, public string $last) {}
public string $fullName {
// Override the "read" action with arbitrary logic.
get => "{$this->first} {$this->last}";
// Override the "write" action with arbitrary logic.
set {
[$this->first, $this->last] = explode(" ", $value, 2);
}
}
}
$user = new User("Scott", "Keck-Warren");
// output:
// Scott Keck-Warren
echo $user->fullName, PHP_EOL;
$user->fullName = "Joe Keck-Warren";
// output:
// Joe
// Keck-Warren
echo $user->first, PHP_EOL;
echo $user->last, PHP_EOL;
There is a potential that this feature could be abused to create some truely hard to debug code. The RFC for property hooks actually states:
A primary use case for hooks is actually to not use them, but retain the ability to do so in the future, should it become necessary.
Want to Know More?
I did a whole article for php[achitect] on property hooks where I got into more details. It's also available in a video version if you're so inclined.